Object-oriented programming Learn web development MDN
Table Of Content
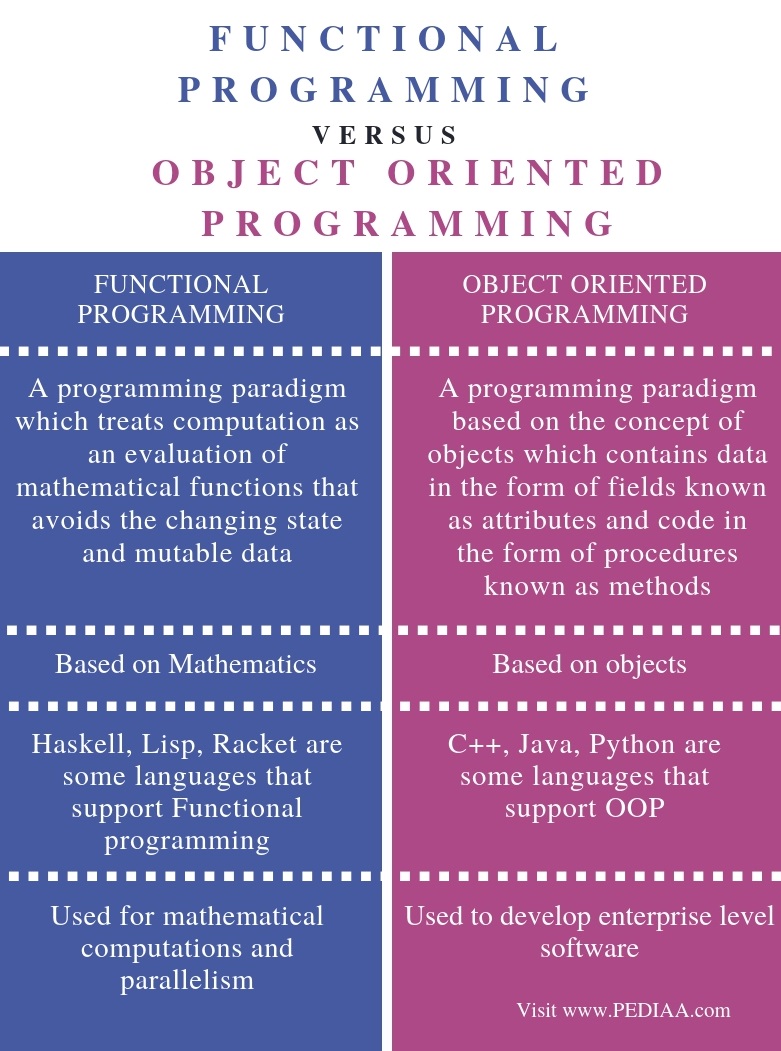
The Adapter Pattern involves a single class called the adapter that is responsible for joining functionalities from independent or incompatible interfaces. For instance, take JavaScript – you can use it for both OOP and functional programming. When you're coding object-oriented JS, you have to think carefully about the structure of the program and plan at the start of coding.
Advance your career with an online degree
Understanding dunder methods is an important part of mastering object-oriented programming in Python, but for your first exploration of the topic, you’ll stick with these two dunder methods. Going hand in hand with adaptability is the desire that software is reusable, that is, code should be usable as a component of different systems in various applications. Developing quality software can be an expensive enterprise, and its cost can be offset somewhat if the software is designed in a way that makes it easily reusable in future applications.
Methods
After doing the dog park example in the previous section, you’ve removed .breed again. You’ll now write code to keep track of a dog’s breed using child classes instead. You can simplify the experience of working with the Dog class by creating a child class for each breed of dog. This allows you to extend the functionality that each child class inherits, including specifying a default argument for .speak(). Methods like .__init__() and .__str__() are called dunder methods because they begin and end with double underscores. There are many dunder methods that you can use to customize classes in Python.
Gang of Four design patterns
Instance methods are functions that you define inside a class and can only call on an instance of that class. Just like .__init__(), an instance method always takes self as its first parameter. Use class attributes to define properties that should have the same value for every class instance. Use instance attributes for properties that vary from one instance to another. You can give .__init__() any number of parameters, but the first parameter will always be a variable called self. When you create a new class instance, then Python automatically passes the instance to the self parameter in .__init__() so that Python can define the new attributes on the object.
Now let's create the invoice class which will contain the logic for creating the invoice and calculating the total price. For now, assume that our bookstore only sells books and nothing else. We will look at the code for a simple bookstore invoice program as an example. But if the SRP is followed, fewer conflicts will appear – files will have a single reason to change, and conflicts that do exist will be easier to resolve. For example, say we have a persistence class that handles database operations, and we see a change in that file in the GitHub commits.

You may think that we could just create multiple classes without an interface and add a save method to all of them. But how are we going to add new functionality without touching the class, you may ask. It is usually done with the help of interfaces and abstract classes. Therefore, it is not a surprise that all these concepts of clean coding, object-oriented architecture, and design patterns are somehow connected and complementary to each other.
Classes and instances

We create 2 classes, InvoicePrinter and InvoicePersistence, and move the methods. We can create new classes for our printing and persistence logic so we will no longer need to modify the invoice class for those purposes. Then we are going to get into the nitty-gritty details – the why's and how's of each principle – by creating a class design and improving it step by step. If you plan on becoming a Cloud engineer, you will need to know at least one programming language. This new Tiger class has all the methods and attributes of the original Cat class but also has a huntGazelle() method. EdX offers interactive online classes and MOOCs from the world’s best universities, including MIT, Harvard, Berkeley, University of Texas, and many others.
Attributes
What is a method (in object-oriented programming)? – TechTarget Definition - TechTarget
What is a method (in object-oriented programming)? – TechTarget Definition.
Posted: Thu, 07 Apr 2022 05:00:12 GMT [source]
It's available inPDF/ePUB/MOBI formats and includes thearchive with code examples inJava, C#, C++, PHP, Python, Ruby,Go, Swift, & TypeScript. Patterns are a toolkit of solutions to commonproblems in software design. They definea common language that helps your teamcommunicate more efficiently. This evolution is crucial because it shapes the analysis model into a detailed design model, essentially serving as a blueprint for constructing the software. You give "Bark" as the default value to the sound parameter in GoldenRetriever.speak().
OOP Design: Composible objects support change - hackernoon.com
OOP Design: Composible objects support change.
Posted: Tue, 12 Dec 2017 08:00:00 GMT [source]
This article outlines the best practices and design patterns for mastering object-oriented programming. A class is a blueprint or a user-defined data type that defines the structure and behavior of objects. Classes encapsulate data (attributes) and the methods (functions) that operate on that data.
Inheritance is the process by which one class takes on the attributes and methods of another. Newly formed classes are called child classes, and the classes that you derive child classes from are called parent classes. Classes define functions called methods, which identify the behaviors and actions that an object created from the class can perform with its data.
In contrast, many folks who have experience with computers initially think there's something strange about object oriented systems. It's interesting that, generations later, the concept of organizing your code into meaningful objects that model the parts of your problem continues to puzzle programmers. With the right tools and resources at your disposal, you can become a master of object-oriented programming and build amazing software that solves real-world problems. By showing only certain data and allowing access and modification through classes and methods, data is protected from exposure. Continuing with the house metaphor, you wouldn't want to expose electrical wires or plumbing to the residents. Finally, we create an instance of the IronMan class and call the attack() method with different types of arguments to demonstrate method overloading.
Comments
Post a Comment